Throwing a javelin and finding where it lands
As part of tutoring physics and maths to high school students, I sometimes write up deep-dive explanations of questions arising during lessons. Below I discuss the example of throwing a javelin, working out where it will hit the ground, and what its highest point will be.

Image credits: Mantee via Clean Png.
We’ve been given the following problem to solve:
The flight path of a javelin (excluding the effects of air resistance) can be described by the function \(f(x) = -0.0265 x^2 + x + 2\), where \(x\) describes the horizontal distance (in metres) from the point where the javelin was thrown and \(f(x)\) describes the height of the javelin above the ground (also in metres).
- a) determine the point at which the javelin hits the ground.
- b) determine the highest point of the javelin’s path.
- c) determine the distance and highest point when the path is described by \(f(x) = -0.03 x^2 + x + 2\).
Let’s begin by visualising the function.
Plotting the function
Plotting the curve corresponding to a function is a good way to build an intuition about the problem at hand. Computers are good at plotting data, so let’s use some Python code in a Jupyter notebook to plot the function \(f(x)\).
Start a Jupyter notebook and enter the following code into it.
import numpy as np # import the numerical libraries and give them the name "np"
import matplotlib.pyplot as plt # import the plotting libraries and give them the name "plt"
# define the function we want to plot
# this uses the general form of a parabola: a x^2 + b x + c
def f(x, a=-0.02625, b=1, c=2):
return a*x**2 + b*x + c
# define a range of x points (which we call "xs" here) from 0 to 50 metres in steps of 0.1 m
xs = np.arange(0, 50, 0.1)
# determine the values on the y-axis from the function f(x) which we defined above (which we call "fs" here)
fs = [f(x) for x in xs]
fig, ax = plt.subplots() # set up the plot
# set the axes labels and plot title
ax.set_xlabel('x (m)')
ax.set_ylabel('f(x) (m)')
ax.set_title('A javelin throw')
# plot the data
ax.plot(xs, fs)
# add a grid to the plot to more easily see the function's values
plt.grid()
# show the plot
plt.show()
Running this code gives the following plot:
Try changing some of the values within your Jupyter notebook and then select “Run selected cell” from the “Run” menu to see how the function’s shape changes.
Note that I guessed the range of \(x\) values; it was clear from the question that the values should start at 0, but the value of 50 was a plain guess.
Now that we’ve plotted the function, some things become more obvious. The javelin is thrown from some initial non-zero height above the ground (i.e. the curve doesn’t cross the \(y\)-axis at 0). This makes sense because someone throwing the javelin will be holding it in their hand above their head and this will be some positive value. We can work out exactly what this value is by setting \(x = 0\) (the javelin’s starting point) in the equation for the function:
\[f(0) = -0.02625 \cdot 0^2 + 0 + 2 = 2 m\]In other words, the javelin is held 2 m above the ground when it is let go.
The curve gives us more information: it crosses the \(x\)-axis (where \(y = 0\)) at approximately 40 m distance. This gives us an estimate of the answer for part (a) of the question. The negative “height” values in the plot are because I chose \(x\) values from 0 to 50 and from roughly 40 m up to 50 m the function is negative. Clearly this this isn’t a physically logical situation (I mean, the javelin would have had to hit the ground very hard for it to go almost 15 m below ground level!), so we can ignore the negative \(f(x)\) values in this upper range.
One thing that the plot hints at but doesn’t explicitly display is that we could extend the curve in the negative \(x\) direction to see where the javelin might have come from had it started its trajectory from ground level. After all, we can equally well put negative \(x\) values into \(f(x)\) as we can positive \(x\) values.
Now that we’ve got some intuition about the problem at hand, let’s start solving things exactly.
Determining the point at which the javelin hits the ground
There are two equivalent ways to solve this problem. Which path you choose depends upon where you live.
Honestly! Let me explain.
In English-speaking countries, it is common to use the quadratic formula to find the roots of a quadratic equation (which is what we need to do here; see later). I live in Germany, and the quadratic formula isn’t taught in schools here. Instead, high-school students are taught the p-q formula, which amounts to the same thing as the quadratic formula. The p-q formula results from solving the reduced quadratic equation for reasons that I will explain below. For completeness, I’m going to mention both methods here. Let’s call the two root-finding methods “the English way” and “the German way” respectively.
“The English way”
If we rewrite the function \(f(x)\) in its general form, we have
\[f(x) = a x^2 + b x + c\]In the case we’re discussing here, the parameters \(a\), \(b\) and \(c\) are: \(a = -0.02625\), \(b = 1\) and \(c = 2\).
Note that the coefficient \(a\) is negative: this means that as \(x\) increases, the parabola rises to a maximum and then decreases again. Because mathematicians love giving such concepts names, this is called a concave function. A positive value of \(a\) would make the function curve downwards to a minimum and then curve upwards again (which is then called a convex function). Try changing the value of \(a\) in the Python code you entered into your Jupyter notebook above to see what effect changing its sign has.
To find out where the javelin hits the ground, we need to work out when \(f(x)\) is equal to zero (i.e. at ground level). This is the same thing as working out where the curve from the plot above crosses the \(x\)-axis. Note that because the function is a parabola (i.e. a polynomial of degree 2) this will happen in two places: one at the end of the javelin’s path of flight and one behind where the javelin was let go. Note that a function merely models a physical situation, it doesn’t have to always match physical reality. After all, one doesn’t talk about negative distances on a day-to-day basis. Remember: all models are wrong, but some models are useful).
Finding where a function crosses the \(x\)-axis is also called finding the roots of a function where a root is a value of \(x\) where \(f(x) = 0\).
Because the function \(f(x)\) is a polynomial of degree 2 (its largest exponential is a 2) this is also called a quadratic equation. There is a known solution for the roots of a quadratic equation which is (confusingly, I find) known as the quadratic formula:
\[x = \frac{-b \pm \sqrt{b^2 - 4ac}}{2a}\]This formula gives us two values, one of which is where the javelin hits the ground. Let’s call the two values \(x_1\) and \(x_2\) respectively:
\[x_1 = \frac{-b + \sqrt{b^2 - 4ac}}{2a}\]and
\[x_2 = \frac{-b - \sqrt{b^2 - 4ac}}{2a}\]We can plug our \(a\), \(b\) and \(c\) values into this equation within our Jupyter notebook. Doing so, we get code like this:
a = -0.02625
b = 1
c = 2
x1 = (-b + np.sqrt(b**2 - 4*a*c))/(2*a)
x2 = (-b - np.sqrt(b**2 - 4*a*c))/(2*a)
print(f"x_1 = {x1:.3f} m and x_2 = {x2:.3f} m")
Running the code gives this output:
x_1 = -1.905 m and x_2 = 40.000 m
In other words, the javelin hit the ground after 40 m. The value for \(x_1\) is negative which tells us that it’s a position behind the person throwing the javelin. We can disregard this solution because it doesn’t make physical sense. The javelin thrower didn’t throw the javelin backwards as well as forwards.
“The German way”
As mentioned earlier, high-school students solve this kind of problem differently in Germany. They use what is called the p-q formula which we get by solving the reduced quadratic equation. Why the reduced quadratic equation? Well, if we can make the factor of the quadratic term (i.e. the \(x^2\) term) equal 1, then we only need to worry about the factor of the linear term (i.e. the \(x\) term) and the constant (the term without any \(x\)-related part). This means we only need to consider two parameters when calculating the zeros of a quadratic equation instead of three parameters. This might not sound like much of a win, but I guess it helps. I’ll show you what I mean.
Let’s take the general form of a quadratic function:
\[f(x) = a x^2 + b x + c\]we know that we want to find solutions to this equation when it is equal to zero, i.e.
\[a x^2 + b x + c = 0\]We can simplify things a little bit by dividing both sides of this equation by \(a\):
\[x^2 + \frac{b}{a} x + \frac{c}{a} = 0\]Hrm, those factors of \(\frac{b}{a}\) and \(\frac{c}{a}\) look complicated. Let’s rename them. Let’s set \(\frac{b}{a} = p\) and \(\frac{c}{a} = q\). Substituting these values into the equation above we get
\[x^2 + p x + q = 0\]which is known as the reduced quadratic equation because we reduced the number of parameters from 3 to 2. The solution to this new form of the quadratic equation is
\[x = -\frac{p}{2} \pm \sqrt{\left(\frac{p}{2}\right)^2 - q}\]and this is the famous p-q formula. This formula is so famous in Germany, that there’s even a song about it.
Let’s stop talking about this thing and use it. Remember that the function we’ve been given is
\[f(x) = -0.02625 x^2 + x + 2\]This isn’t in a form we can use for the p-q formula. Remember that we want to find the points where this function is equal to zero, hence we can rewrite this as
\[-0.02625 x^2 + x + 2 = 0\]We can now divide both sides by \(-0.02625\) to get
\[x^2 - \frac{x}{0.02625} - \frac{2}{0.02625} = 0\]This equation is now in the form we need for the p-q formula, where \(p = -\frac{1}{0.02625}\) and \(q = -\frac{2}{0.02625}\). Plugging these values into the formula within our Jupyter notebook we get:
p = -1/0.02625
q = -2/0.02625
x1_pq = -p/2 + np.sqrt((p/2)**2 - q)
x2_pq = -p/2 - np.sqrt((p/2)**2 - q)
print(f"x_1 = {x1_pq:.3f} m and x_2 = {x2_pq:.3f} m")
where I’ve added the suffix _pq
to the x1
and x2
variables to
highlight that these have been calculated via the p-q formula.
Running this code produces this output:
x_1 = 40.000 m and x_2 = -1.905 m
In other words, we get the same result as before when using “the English way”, but this time \(x_1\) is the positive value (and hence where the javelin hits the ground) and \(x_2\) is the negative value (the zero of the function behind the javelin thrower).
I don’t know if this is an easier or clearer way to do things, but it gives the same result. You just have to remember to divide everything by the factor of the quadratic term (i.e. the \(x^2\) bit) before working out what \(p\) and \(q\) are.
Taking the long way round
While explaining this problem to a student recently, I kept having the feeling that there was something special about the value \(0.02625\). I mean, it seemed “clean”, “round” or “regular” in some kind of way; like it could be the square of some number or perhaps it has some kind of special form that wasn’t obvious from the decimal representation. Also, I felt that this number must be special in some way otherwise the distance the javelin was thrown wouldn’t be exactly 40 m. Although I couldn’t spot anything special during the lesson, it kept bugging me. After the lesson, I worked out what was special and I worked out that it wasn’t necessary to use a calculator (or Python) to work out how far the javelin flew.
What was special about the number was that it can be written as a fraction. I.e. it turns out that
\[0.02625 = \frac{21}{800}\]Admittedly, I needed to use a computer algebra system such as Sage to find the fraction,1 but once you know what the fraction is, it’s no longer necessary to use a calculator. In the end, the calculation wasn’t that much simpler, but I found it interesting, so let’s look at it.
Our function now looks like this
\[f(x) = -\frac{21}{800} x^2 + x + 2\]in its “fractional form”.
The first thing to note is that it won’t be any fun writing \(\frac{21}{800}\) all over the place, so let’s replace this value with the symbol \(d\). The function is now:
\[f(x) = -d x^2 + x + 2\]We want to find when this function is equal to zero, i.e. we want to solve this equation:
\[-d x^2 + x + 2 = 0\]We also want this in the form required for the p-q formula, so let’s now divide both sides by \(-d\):
\[x^2 - \frac{x}{d} - \frac{2}{d} = 0\]This result means that \(p = -\frac{1}{d}\) and \(q = -\frac{2}{d}\). Plugging these numbers into the p-q formula gives
\[x = \frac{1}{2 d} \pm \sqrt{\left(\frac{-1}{2 d}\right)^2 + \frac{2}{d}}\]Multiplying out the exponent, we get
\[x = \frac{1}{2 d} \pm \sqrt{\frac{1}{4 d^2} + \frac{2}{d}}\]If you squint at this equation, you might notice that the \(4 d^2\) in the denominator under the square root could potentially be factored out. Its square root would give a factor of \(\frac{1}{2d}\) in front of the square root, which is also the first term of the equation. That hints at more possible simplifications. Let’s do that.
First, let’s put the two terms under the square root over a common denominator of \(4 d^2\) by multiplying the second term (i.e. \(\frac{2}{d}\)) by \(\frac{4 d}{4 d}\):
\[x = \frac{1}{2 d} \pm \sqrt{\frac{1}{4 d^2} + \frac{8 d}{4 d^2}}\]This simplifies a bit to:
\[x = \frac{1}{2 d} \pm \sqrt{\frac{1 + 8 d}{4 d^2}}\]which we can now write as
\[x = \frac{1}{2 d} \pm \frac{\sqrt{1 + 8 d}}{\sqrt{4 d^2}}\]As you can see \(\sqrt{4 d^2} = 2d\), hence
\[x = \frac{1}{2 d} \pm \frac{\sqrt{1 + 8 d}}{2 d}\]Extracting the factor of \(\frac{1}{2d}\), we get
\[x = \frac{1}{2 d} \left(1 \pm \sqrt{1 + 8 d}\right)\]That looks a bit easier to handle. Let’s now substitute our value for \(d = \frac{21}{800}\):
\[x = \frac{800}{42} \left(1 \pm \sqrt{1 + \frac{8 \times 21}{800}}\right)\]We can get rid of at least a factor of 8 within the square root to simplify things a bit
\[x = \frac{800}{42} \left(1 \pm \sqrt{1 + \frac{21}{100}}\right)\]Putting the terms under the square root over a common denominator, we get
\[x = \frac{800}{42} \left(1 \pm \sqrt{\frac{100 + 21}{100}}\right)\]which is
\[x = \frac{800}{42} \left(1 \pm \sqrt{\frac{121}{100}}\right)\]The square root of 100 is 10, so we can extract a factor of \(\frac{1}{10}\) from the square root
\[x = \frac{800}{42} \left(1 \pm \frac{1}{10} \sqrt{121}\right)\]Hrm, \(\sqrt{121}\) looks strangely familiar. Hey, it’s just 11 (i.e. \(11 \times 11 = 121\))! That’s cool! We can get rid of that nasty square root now:
\[x = \frac{800}{42} \left(1 \pm \frac{11}{10}\right)\]Using a common denominator again, we have
\[x = \frac{800}{42} \left(\frac{10 \pm 11}{10}\right)\]Cancelling out the factor of 10, we get
\[x = \frac{80}{42} \left(10 \pm 11\right)\]The javelin will have been thrown to the larger of the two solutions, in other words, we can take the \(+\) part of the \(\pm\)
\[x = \frac{80}{42} \left(10 + 11\right)\]which is
\[x = \frac{80}{42} \times 21\]We now see that 42 is twice 21, so this reduces to
\[x = \frac{80}{2}\]which is
\[x = 40\]In other words, the javelin hit the ground after 40 m. Finally! We managed to work out what the value was without a calculator! Ok, so that was a lot of work, but it was somehow satisfying that it wasn’t necessary to use a machine to find the answer.
Determining the highest point of the javelin’s path (method 1)
If we look at the plot of the function we created earlier, we can see that when the javelin reaches its highest point, the slope of its path is completely flat. Up to that point, the slope of the javelin’s path is pointing upwards and after the highest point, the slope of the javelin’s path is pointing downwards. The slope of a curve at a given point is given by its tangent. The tangent to a curve at a given point (and hence the curve’s slope at that point) can be determined by taking the derivative of the function at that point. For our function \(f(x)\), we can take its derivative and thus find the function’s slope at any given point \(x\) along its path.
You will often see the derivative of a function written as \(f'(x)\) or as \(\frac{df}{dx}\); they mean the same thing. To take the derivative of a power such as \(x^n\), take the value \(n\) and multiply it by the power reduced by 1, i.e. the derivative of \(x^n\) is \(n x^{n-1}\). We can apply this rule to our function \(f(x)\) term-by-term in the expression.
Thus the derivative of
\[f(x) = ax^2 + bx + c\]is
\[f'(x) = 2ax + b\]where we have used the fact that taking the derivative of a constant is zero. A constant doesn’t vary as we change \(x\), hence its slope is always zero and thus its derivative is zero.
Since we know that the tangent to the function is completely flat at the highest point of the javelin’s path, we can say that the slope at this point is zero. Hence we only need to find the value of \(x\) where the derivative is equal to zero. I.e. we need to solve this equation for \(x\):
\[f'(x) = 0 = 2ax + b\]Subtracting \(b\) from both sides of the equation, we get
\[-b = 2ax\]and dividing both sides of the equation by \(2a\) we find the value of \(x\) when the javelin is at its highest point:
\[x = \frac{-b}{2a}\]We can do this calculation in our Jupyter notebook by plugging in the values for \(a\) and \(b\) like so:
a = -0.02625
b = 1
x = -b/(2*a)
print(f"x at maximum height is {x:.3f} m")
Running this code gives this output:
x at maximum height is 19.048 m
Does this value make sense? It certainly looks like the highest point occurs at a horizontal distance slightly less than 20 m in the plot of the function we made above. So that’s a good indicator that our answer makes sense.
Also, we found that the javelin hit the ground 40 m. It makes sense that the horizontal distance corresponding to the highest point would be at approximately half that value, i.e. it should be approximately 20 m. Why do we expect the highest point to be at approximately half the thrown distance? Well, a parabola is symmetric across its maximum (or minimum if it is convex). So, if the javelin had been thrown from the point (0, 0) (i.e. from ground level) its highest point would be halfway to where it hit the ground. We expect this distance to be a little bit smaller than 20 m because the javelin is thrown from a height of 2 m above the ground. In a sense, it was already part of the way to its destination when thrown. In other words, yes, the \(x\) distance corresponding to the maximum height of the curve does make sense.
To find the height of the highest point of the javelin’s path, we plug the value we got for the horizontal distance travelled to the javelin’s highest point into our function. I.e., we substitute 19.048 for \(x\) into the function we’re initially given.
\[f(19.048) = -0.02625 \cdot 19.048^2 + 19.048 + 2\]Converting this into Python within our Jupyter notebook, we have:
print(f"Maximum height is {-0.0265*19.048**2 + 19.048 + 2:.3f} m.")
and when we run this, we get:
Maximum height is 11.433 m.
Therefore the javelin’s maximum height was 11.433 m. Looking back at our plot of the function from earlier we see that the maximum is slightly above 10 and is probably some value between 11 and 12, which matches the result we calculated here. Thus we can be confident that the value we calculated is correct.
Determining the highest point of the javelin’s path (method 2)
We can work out the horizontal distance corresponding to the javelin’s highest point another way. An important point to realise is that a parabola is symmetric along the \(x\)-axis. I.e. half the function lies on one side of the highest point and the other half lies on the other side of the highest point. We can use this property as well as the roots of the equation that we calculated earlier to find the highest point of the javelin’s trajectory. And now we see that it was helpful to calculate the root of the equation behind the thrower!
Using a symmetry of the problem, we now know that the highest point of the javelin’s path is halfway between the two roots. We can calculate the distance between the two roots using our Jupyter notebook by entering the following code.
print(f"The distance between the two roots is: x₂ - x₁ = {x2 - x1:.3f} m.")
where we use the x1
and x2
values we calculated from “the English way”
above.
Running this, we get the following output:
The distance between the two roots is: x₂ - x₁ = 41.905 m.
The location of the highest point will be half the distance between the two roots plus the position of the point behind the thrower. I.e.:
\[\mathrm{highest\ point} = x_1 + \frac{x_2 - x_1}{2}\]In other words, we start at \(x_1\) and move forward half of the distance between the two zeros of the function.
We can calculate this in the Jupyter notebook by entering the following code and running it:
print(f"x at maximum height is {(x2 - x1)/2 + x1:.3f} m")
Its output is:
x at maximum height is 19.048 m
which matches the value we got by taking the derivative and finding the point where the slope was equal to zero. Again, since this is the value of \(x\) at the highest point of the javelin’s path, we just need to plug this value into our function.
\[f(19.048) = -0.02625 \cdot 19.048^2 + 19.048 + 2\]Running the calculation in our Jupyter notebook:
print(f"Maximum height is {-0.0265*19.048**2 + 19.048 + 2:.3f} m.")
gives this output:
Maximum height is 11.433 m.
Which is the result we got earlier. It’s reassuring that by taking different paths we arrive at the same result!
Equivalently, the horizontal distance at the javelin’s highest point will be the position where the javelin hit the ground minus half the distance between the two roots. Using this version of the calculation, we get the same result as above:
\[\mathrm{highest\ point} = x_2 - \frac{x_2 - x_1}{2}\]where we start at the point \(x_2\) and move backwards half of the distance between the two zeros of the function. In Python:
print(f"x at maximum height is {x2 - (x2 - x1)/2:.3f} m")
gives this output:
x at maximum height is 19.048 m
and equals the two other results for this value that we computed previously. Fairly obviously, if we then plug this value into the function, we get the highest height the javelin reaches, i.e. 11.433 m.
Determining the distance and highest point when the path is described by \(f(x)=−0.03x^2+x+2\)
This is a very similar question to which we already have an answer. Because of this similarity, it makes sense to compare the two functions with one another:
\[\begin{align} f_1(x) &= -0.0265 x^2 + x + 2\\ f_2(x) &= -0.03 x^2 + x + 2 \end{align}\]where we’ve labelled the functions according to the order in which they were presented to us in the question.
We can see straight away that there’s only one difference between the two functions: the \(a\) coefficient is a bit more negative in \(f_2(x)\). What does this tell us about the values the function will take? Because the quadratic term has a more negative coefficient, we would expect the height to be lower than in the initial example and the distance to be less. Why? Well, the more negative value will “put pressure” on the function to come down sooner than the function in the first part of the exercise.
Let’s plot the function to see if our intuition is correct. Enter the following code into your Jupyter notebook
# we've already imported the numerical and plotting libraries earlier, hence we don't need to do this again
# we've also defined the function to plot in a general way earlier;
# we just need to specify the "a" value when generating the list of "fs" this time
# define a shorter list of x points from 0 to 40 metres in steps of 0.1 m
xs = np.arange(0, 40, 0.1)
# determine the values on the y-axis from the function f(x) with the updated "a" value
fs = [f(x, a=-0.03) for x in xs]
fig, ax = plt.subplots() # set up the plot
# set the axes labels and plot title
ax.set_xlabel('$x$ (m)')
ax.set_ylabel('$f_2(x)$ (m)')
ax.set_title('A second javelin throw')
# plot the data
ax.plot(xs, fs)
# add a grid to the plot to more easily see the function's values
plt.grid()
# show the plot
plt.show()
and run it. You should see something like this:
This result matches our intuition: the distance thrown seems to be approximately 35 m this time; less than the distance from part (a). The highest point looks to occur at a horizontal distance of approximately 17 m, also slightly less than our answer from part (a).
Let’s work out the distance travelled using both the “English” and “German” ways.
Finding the distance the “English” way
Using the quadratic formula again to work out at which \(x\) values \(f_2(x)\) is zero, we have:
\[x = \frac{-b \pm \sqrt{b^2 - 4ac}}{2a}\]Plugging the appropriate values for \(a\), \(b\) and \(c\) into this equation within our Jupyter notebook
a2 = -0.03
b = 1
c = 2
x1_new = (-b + np.sqrt(b**2 - 4*a2*c))/(2*a2)
x2_new = (-b - np.sqrt(b**2 - 4*a2*c))/(2*a2)
print(f"x₁ = {x1_new:.3f} m and x₂ = {x2_new:.3f} m.")
gives this output:
x₁ = -1.893 m and x₂ = 35.226 m.
In other words, the javelin hit the ground after 35.226 m. This result matches our initial guess from the plot of the function. Again, the value for \(x_1\) is negative which tells us that it’s a position behind the person throwing the javelin and is thus a solution that we can disregard as not making physical sense.
Finding the distance the “German” way
To be able to use the p-q formula, we need to take \(f_2(x)\) and consider that we’re finding the \(x\) values when the function is equal to 0. In other words, we need to solve this equation
\[-0.03 x^2 + x + 2 = 0\]To get things into the right form, we need to divide both sides of the equation by -0.03, which gives
\[x^2 - \frac{x}{0.03} - \frac{2}{0.03} = 0\]thus we can see that our values for p and q are: \(p = -\frac{1}{0.03}\) and \(q = -\frac{2}{0.03}\). Remember that the p-q formula is
\[x = -\frac{p}{2} \pm \sqrt{\left(\frac{p}{2}\right)^2 - q}\]We can now plug in the values for \(p\) and \(q\) to find the two roots of \(f_2(x)\) within our Jupyter notebook:
p = -1/0.03
q = -2/0.03
x1 = -p/2 + np.sqrt((p/2)**2 - q)
x2 = -p/2 - np.sqrt((p/2)**2 - q)
print(f"x_1 = {x1:.3f} m and x_2 = {x2:.3f} m")
You should see this output:
x_1 = 35.226 m and x_2 = -1.893 m
which matches the result calculated via the quadratic formula.
The highest point
The highest point can again be found by taking the derivative of the general form of the function and plugging in the values for \(a\) and \(b\):
\[x = \frac{-b}{2a}\]Entering the following code into the Jupyter notebook
a2 = -0.03
b = 1
x_new = -b/(2*a2)
print(f"x at maximum height is {x_new:.3f} m")
where I’ve been careful to use a different variable for \(a\) (a2
) and
\(x\) (x_new
) to avoid clashes with earlier calculations.
Running this code it gives:
x at maximum height is 16.667 m
which is close to the value we guessed from looking at the plotted curve above. This is also less than the \(\approx 19.05\) m we got from part (a), which matches our intuition from the start of this section. This horizontal distance corresponds to where the javelin is at its highest point, thus we substitute this value for \(x\) in
\[f_2(x) = -0.03 x^2 + x + 2\]which is
\[f_2(16.667) = -0.03 \cdot 16.667^2 + 16.667 + 2\]Using Python to do the hard work for us
print(f"Maximum height is {-0.03*16.667**2 + 16.667 + 2:.3f} m.")
we get
Maximum height is 10.333 m.
This means that the javelin was thrown a bit lower than the javelin in the initial part of the question. This value matches the maximum of the curve in the plot above as well as our initial intuition of the situation and thus we can be confident that our calculated value is correct.
What did we learn?
So, after having gone through all this work, what did we learn?
We found out that:
- there’s often more than one way to solve a problem in maths. It’s usually a good idea to follow multiple paths to a solution; a second result will verify an initial answer.
- we can use the inherent symmetry of a problem and a bit of logic to find a solution. Using the symmetry of a quadratic function as well as its roots we were able to find the highest point of the javelin’s path.
- we can use calculus to find the highest (or lowest) points of a function. These points are where the slope (and hence the derivative) of the function are zero. In the example here, this information allowed us to find the highest point of the javelin’s path.
- sometimes the common path to a solution is cultural. In the case here, either you use the quadratic formula or the p-q formula. It doesn’t matter which: both paths lead to the same result.
- it’s not always necessary to use a calculator. Solving things by hand does work and is a useful skill to develop.
That’s it! Happy problem-solving!
-
Found via the
simplest_rational()
method. I.e.0.02625.simplest_rational()
. ↩
Support
If you liked this post and want to see more like this, please buy me a coffee!
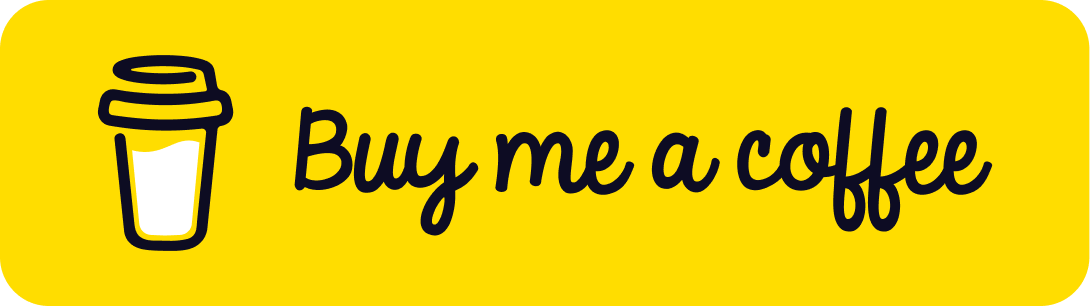